Building a Simple Calculator with JavaScript
Published June 27, 2024 at 5:52 pm
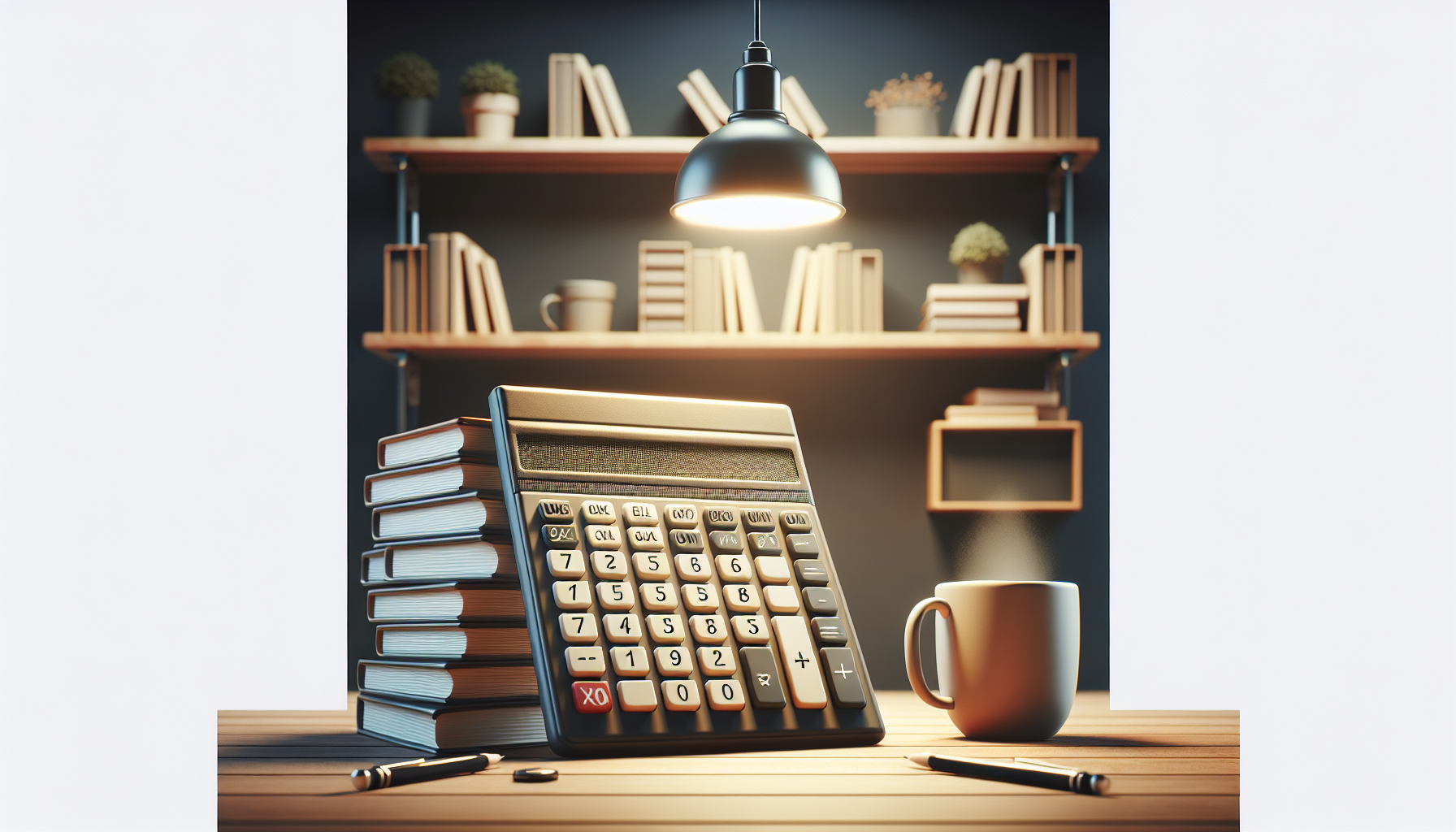
Introduction
Building a simple calculator with JavaScript is a great project for beginners who are learning the basics of web development.
It allows you to understand how HTML, CSS, and JavaScript can work together to create interactive web applications.
Let’s dive into creating a basic calculator that performs addition, subtraction, multiplication, and division.
TL;DR: How Do You Build a Simple Calculator with JavaScript?
Create an HTML structure with buttons for numbers and operations.
Add a text area to display the input and output.
Use JavaScript to add event listeners to the buttons and perform calculations when they are clicked.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple JavaScript Calculator</title>
<style>
.calculator { width: 200px; margin: 100px auto; }
.display { width: 100%; height: 40px; margin-bottom: 10px; text-align: right; padding: 10px; box-sizing: border-box; }
.button { width: 50px; height: 50px; margin: 5px; }
</style>
</head>
<body>
<div class="calculator">
<input type="text" class="display" disabled id="display" />
<button class="button" onclick="appendValue('1')">1</button>
<button class="button" onclick="appendValue('2')">2</button>
<button class="button" onclick="appendValue('3')">3</button>
<button class="button" onclick="performOperation('+')">+</button>
<button class="button" onclick="appendValue('4')">4</button>
<button class="button" onclick="appendValue('5')">5</button>
<button class="button" onclick="appendValue('6')">6</button>
<button class="button" onclick="performOperation('-')">-</button>
<button class="button" onclick="appendValue('7')">7</button>
<button class="button" onclick="appendValue('8')">8</button>
<button class="button" onclick="appendValue('9')">9</button>
<button class="button" onclick="performOperation('*')">×</button>
<button class="button" onclick="appendValue('0')">0</button>
<button class="button" onclick="calculate()">=</button>
<button class="button" onclick="clearDisplay()">C</button>
<button class="button" onclick="performOperation('/')">÷</button>
</div>
<script>
let display = document.getElementById('display');
let currentInput = '';
let currentOperation = null;
let previousInput = '';
function appendValue(value) {
currentInput += value;
display.value = currentInput;
}
function performOperation(operation) {
previousInput = currentInput;
currentInput = '';
currentOperation = operation;
}
function calculate() {
if (currentOperation && previousInput && currentInput) {
let result;
switch (currentOperation) {
case '+':
result = parseFloat(previousInput) + parseFloat(currentInput);
break;
case '-':
result = parseFloat(previousInput) - parseFloat(currentInput);
break;
case '*':
result = parseFloat(previousInput) * parseFloat(currentInput);
break;
case '/':
result = parseFloat(previousInput) / parseFloat(currentInput);
break;
}
display.value = result;
currentInput = result.toString();
previousInput = '';
currentOperation = null;
}
}
function clearDisplay() {
currentInput = '';
previousInput = '';
currentOperation = null;
display.value = '';
}
</script>
</body>
</html>
Creating the HTML Structure
Start with an HTML file to create the basic structure of the calculator.
Create a div
element with a class “calculator”.
Add an input
element inside the div
to serve as the display of the calculator.
Adding Buttons for Numbers and Operations
Add buttons for numbers 0-9.
You’ll also need buttons for the operations: addition, subtraction, multiplication, and division.
Include an equals button to calculate the result and a clear button to reset the display.
Styling the Calculator with CSS
Use CSS to style the calculator.
Give it a width and center it on the page.
Style the buttons and display area to make them more attractive.
Implementing JavaScript to Handle Events
Write JavaScript functions to handle the events for clicking the buttons.
Use document.getElementById()
to reference the display element.
Append the clicked number to the current input when a number button is clicked.
Store the current input and operation when an operation button is clicked.
Perform the calculation and display the result when the equals button is clicked.
Handling Various Operations
Handle different operations like addition, subtraction, multiplication, and division using switch
statements.
Use parseFloat()
to convert string inputs to numbers for calculations.
Adding Error Handling
Add checks to prevent errors like dividing by zero.
Update the display to show an error message if an invalid operation is performed.
Fine-Tuning UX and UI
Ensure the calculator is user-friendly by making buttons large enough to click easily.
Use consistent styling for a clean look.
Test the calculator on different devices to ensure it works well on all screen sizes.
Example of Complete HTML, CSS, and JavaScript
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple JavaScript Calculator</title>
<style>
.calculator { width: 200px; margin: 100px auto; }
.display { width: 100%; height: 40px; margin-bottom: 10px; text-align: right; padding: 10px; box-sizing: border-box; }
.button { width: 50px; height: 50px; margin: 5px; }
</style>
</head>
<body>
<div class="calculator">
<input type="text" class="display" disabled id="display" />
<button class="button" onclick="appendValue('1')">1</button>
<button class="button" onclick="appendValue('2')">2</button>
<button class="button" onclick="appendValue('3')">3</button>
<button class="button" onclick="performOperation('+')">+</button>
<button class="button" onclick="appendValue('4')">4</button>
<button class="button" onclick="appendValue('5')">5</button>
<button class="button" onclick="appendValue('6')">6</button>
<button class="button" onclick="performOperation('-')">-</button>
<button class="button" onclick="appendValue('7')">7</button>
<button class="button" onclick="appendValue('8')">8</button>
<button class="button" onclick="appendValue('9')">9</button>
<button class="button" onclick="performOperation('*')">×</button>
<button class="button" onclick="appendValue('0')">0</button>
<button class="button" onclick="calculate()">=</button>
<button class="button" onclick="clearDisplay()">C</button>
<button class="button" onclick="performOperation('/')">÷</button>
</div>
<script>
let display = document.getElementById('display');
let currentInput = '';
let currentOperation = null;
let previousInput = '';
function appendValue(value) {
currentInput += value;
display.value = currentInput;
}
function performOperation(operation) {
previousInput = currentInput;
currentInput = '';
currentOperation = operation;
}
function calculate() {
if (currentOperation && previousInput && currentInput) {
let result;
switch (currentOperation) {
case '+':
result = parseFloat(previousInput) + parseFloat(currentInput);
break;
case '-':
result = parseFloat(previousInput) - parseFloat(currentInput);
break;
case '*':
result = parseFloat(previousInput) * parseFloat(currentInput);
break;
case '/':
result = parseFloat(previousInput) / parseFloat(currentInput);
break;
}
display.value = result;
currentInput = result.toString();
previousInput = '';
currentOperation = null;
}
}
function clearDisplay() {
currentInput = '';
previousInput = '';
currentOperation = null;
display.value = '';
}
</script>
</body>
</html>
Frequently Asked Questions
What is the basic structure of an HTML calculator?
A basic HTML calculator consists of a display area and buttons for numbers and operations.
Can I add more complex functions to this calculator?
Yes, you can add functions like square roots, percentages, and more by extending the JavaScript code.
How can I make this calculator responsive?
Use CSS media queries to adjust button sizes and layout for different screen sizes.
Creating a Functioning Calculator with JavaScript
Now that you’ve seen the basic structure, let’s deepen our understanding of each part of the calculator.
This section will break down the HTML, CSS, and JavaScript that make the calculator functional.
Step-by-Step Breakdown of HTML Structure
The core of your calculator lies in its HTML structure.
Each button in the calculator is created within a div
element class named “calculator”.
The input
element acts as the display screen of the calculator.
Buttons are created for numbers (0-9), arithmetic operations (+, -, *, /), a clear button (C), and an equals button (=).
Here’s the code snippet for the HTML structure.
<div class="calculator">
<input type="text" class="display" disabled id="display" />
<button class="button" onclick="appendValue('1')">1</button>
<button class="button" onclick="appendValue('2')">2</button>
<button class="button" onclick="appendValue('3')">3</button>
<button class="button" onclick="performOperation('+')">+</button>
<button class="button" onclick="appendValue('4')">4</button>
<button class="button" onclick="appendValue('5')">5</button>
<button class="button" onclick="appendValue('6')">6</button>
<button class="button" onclick="performOperation('-')">-</button>
<button class="button" onclick="appendValue('7')">7</button>
<button class="button" onclick="appendValue('8')">8</button>
<button class="button" onclick="appendValue('9')">9</button>
<button class="button" onclick="performOperation('*')">×</button>
<button class="button" onclick="appendValue('0')">0</button>
<button class="button" onclick="calculate()">=</button>
<button class="button" onclick="clearDisplay()">C</button>
<button class="button" onclick="performOperation('/')">÷</button>
</div>
Applying CSS to Style the Calculator
The aesthetics of your calculator play a significant role in user experience.
CSS gives a width to the calculator and centers it on the page.
The display area is adjusted to have a specific height, and text is right-aligned.
Buttons are given a width and height, and margins are applied for better spacing.
Here’s how you can style your calculator:
<style>
.calculator { width: 200px; margin: 100px auto; }
.display { width: 100%; height: 40px; margin-bottom: 10px; text-align: right; padding: 10px; box-sizing: border-box; }
.button { width: 50px; height: 50px; margin: 5px; }
</style>
JavaScript Functions for Event Handling
JavaScript is the engine that makes your calculator interact and perform calculations.
Using document.getElementById()
, you reference the display element to update it with user input and results.
Event listeners are added to each button to capture user actions like entering numbers and performing arithmetic operations.
Here’s a closer look at the JavaScript code:
<script>
let display = document.getElementById('display');
let currentInput = '';
let currentOperation = null;
let previousInput = '';
// Append value to the display
function appendValue(value) {
currentInput += value;
display.value = currentInput;
}
// Store value and operation
function performOperation(operation) {
previousInput = currentInput;
currentInput = '';
currentOperation = operation;
}
// Calculate result
function calculate() {
if (currentOperation && previousInput && currentInput) {
let result;
switch (currentOperation) {
case '+':
result = parseFloat(previousInput) + parseFloat(currentInput);
break;
case '-':
result = parseFloat(previousInput) - parseFloat(currentInput);
break;
case '*':
result = parseFloat(previousInput) * parseFloat(currentInput);
break;
case '/':
if(currentInput === '0') {
display.value = 'Error';
return;
}
result = parseFloat(previousInput) / parseFloat(currentInput);
break;
}
display.value = result;
currentInput = result.toString();
previousInput = '';
currentOperation = null;
}
}
// Clear display
function clearDisplay() {
currentInput = '';
previousInput = '';
currentOperation = null;
display.value = '';
}
</script>
Enhancing User Experience
To make the calculator more user-friendly, ensure that buttons are large enough for easy clicking.
Consistent styling enhances the visual appeal.
Test the calculator on various devices to ensure compatibility and responsiveness.
Extending Functionality with Additional Features
While the basic calculator is functional, there is always room for improvement.
Adding features like square root, percentages, or memory functions can enhance the utility of the calculator.
Here’s an example of how to add a square root function:
function performOperation(operation) {
if (operation === 'sqrt') {
currentInput = Math.sqrt(parseFloat(currentInput)).toString();
display.value = currentInput;
} else {
previousInput = currentInput;
currentInput = '';
currentOperation = operation;
}
}
Extend the HTML to include the new button:
<button class="button" onclick="performOperation('sqrt')">&sqrt;</button>
FAQs
How can I add more complex operations like exponents?
You can use JavaScript’s Math.pow()
function to add exponentiation.
What if the calculator doesn’t work on a specific browser?
Ensure that your JavaScript code is compatible with that browser. Test on multiple browsers to identify the issue.
Can I style the buttons differently for better UX?
Yes, you can use CSS to customize the button styles to make them more user-friendly.
Shop more on Amazon