JavaScript and the Clipboard API: Copy and Paste
Published June 5, 2024 at 5:50 pm
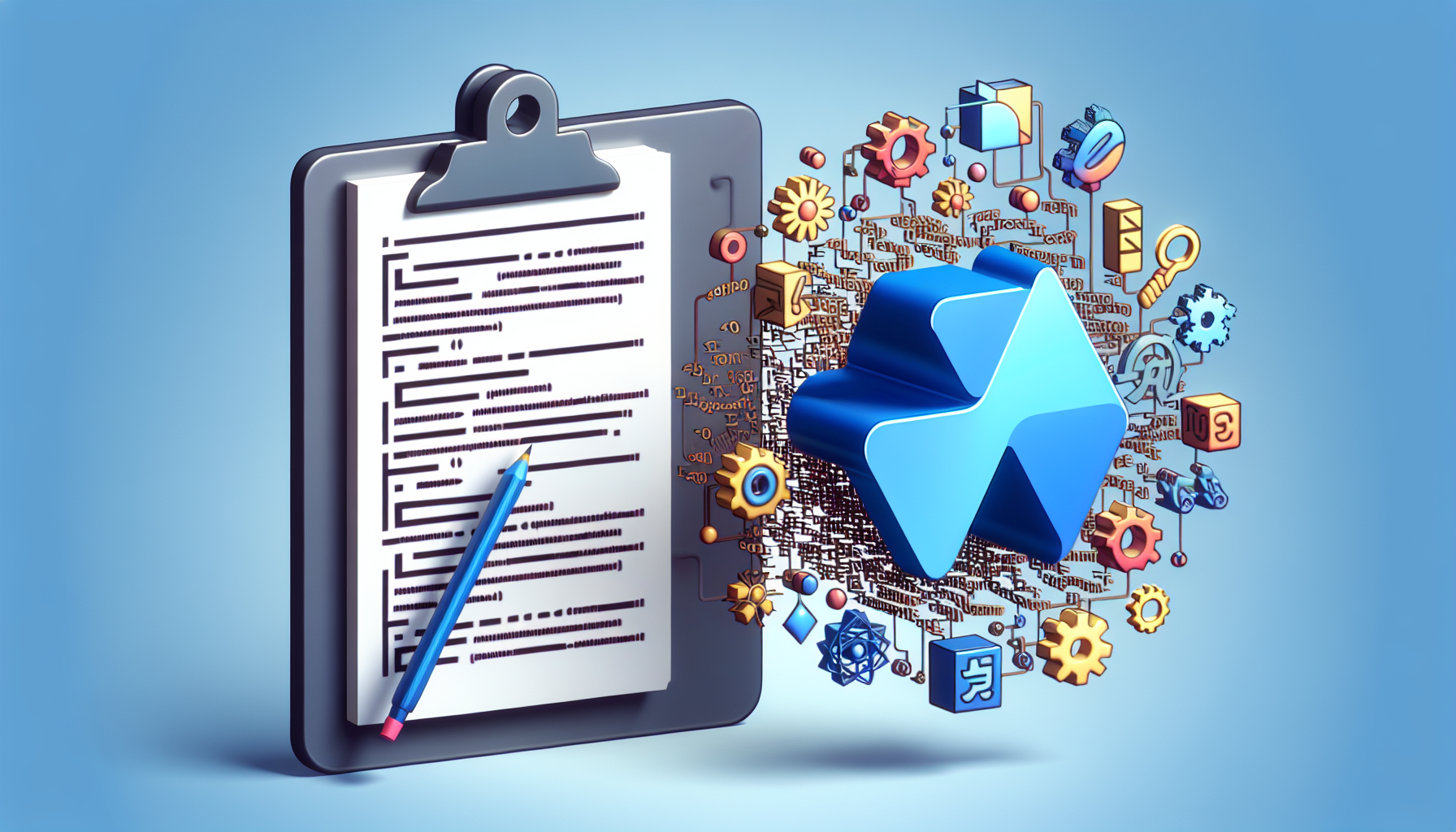
Introduction to JavaScript and the Clipboard API
Using the Clipboard API in JavaScript can simplify the process of copying and pasting content programmatically.
The Clipboard API provides a way to interact with the system clipboard in a secure and consistent manner.
This guide will walk you through everything you need to know about using the Clipboard API in JavaScript.
TLDR: How to Use the Clipboard API in JavaScript
To copy text to the clipboard:
// Copy text to the clipboard
navigator.clipboard.writeText('Text to copy').then(function() {
console.log('Text successfully copied to clipboard.');
}, function(err) {
console.error('Could not copy text:', err);
});
To read text from the clipboard:
// Read text from the clipboard
navigator.clipboard.readText().then(function(text) {
console.log('Text read from clipboard:', text);
}, function(err) {
console.error('Could not read text:', err);
});
What is the Clipboard API?
The Clipboard API is a set of asynchronous JavaScript methods designed to interact with the system clipboard.
It ensures your web application can copy, cut, and paste text securely.
Unlike older methods like document.execCommand(), the Clipboard API offers a more modern approach.
It provides greater security and robustness in handling clipboard operations.
Benefits of Using the Clipboard API
The Clipboard API improves security by requiring user interaction for clipboard operations.
It standardizes clipboard interactions across different browsers.
It supports asynchronous operations, leading to better performance and user experience.
Modern web applications can efficiently use the Clipboard API for handling clipboard tasks.
Pros:
- Improved security over older methods.
- Standardized API across browsers.
- Supports asynchronous operations, improving performance.
Cons:
- Limited browser support in older versions.
- Requires user interaction for clipboard access.
How to Copy Text to Clipboard
Copying text to the clipboard using the Clipboard API is straightforward.
The method writeText() is used to write text to the clipboard.
Here’s an example of copying text to the clipboard:
// Function to copy text to clipboard
function copyToClipboard(text) {
navigator.clipboard.writeText(text).then(function() {
console.log('Text copied to clipboard.');
}).catch(function(err) {
console.error('Failed to copy text.', err);
});
}
// Usage
copyToClipboard('Hello, World!');
The above code defines a function to copy text to the clipboard using navigator.clipboard.writeText().
It uses a promise to handle success and error cases.
How to Read Text from Clipboard
Reading text from the clipboard involves using the readText() method.
Here’s an example of reading text from the clipboard:
// Function to read text from clipboard
function readFromClipboard() {
navigator.clipboard.readText().then(function(text) {
console.log('Text read from clipboard:', text);
}).catch(function(err) {
console.error('Failed to read text.', err);
});
}
// Usage
readFromClipboard();
The code reads text from the clipboard and logs it to the console.
It uses navigator.clipboard.readText() and handles success and error conditions with a promise.
Browser Compatibility for the Clipboard API
The Clipboard API is well-supported in modern web browsers.
However, older browsers may not support it, which can be a drawback.
Ensuring your application checks for Clipboard API support is crucial:
// Check for Clipboard API support
if (navigator.clipboard) {
console.log('Clipboard API is supported.');
} else {
console.log('Clipboard API is not supported.');
}
This conditional check informs you if the Clipboard API is available.
Handling Clipboard Permissions
Handling permissions is essential when working with the Clipboard API.
Users must grant clipboard access permissions explicitly.
The Permissions API can check and request permissions:
// Request clipboard-write permission
navigator.permissions.query({ name: 'clipboard-write' }).then(function(permissionStatus) {
console.log('clipboard-write permission state is', permissionStatus.state);
permissionStatus.onchange = function() {
console.log('clipboard-write permission state changed to', this.state);
};
});
The above code requests clipboard-write permission and logs the state.
It also listens for changes in the permission state.
Common Issues and Their Solutions
Using the Clipboard API can sometimes present challenges.
Being aware of common issues and their solutions can be helpful.
Issue: Clipboard API not supported
Check if the Clipboard API is available using navigator.clipboard.
If not, consider using a fallback method.
Issue: Permission denied
Ensure your site is served over HTTPS as Clipboard API requires a secure context.
Request the necessary permissions using the Permissions API.
User interaction is required for clipboard operations.
Issue: Clipboard read/write fails
Use promises to handle success and error cases appropriately.
Ensure you catch and handle errors gracefully.
Frequently Asked Questions
How do I copy text in JavaScript without the Clipboard API?
You can use document.execCommand(‘copy’) but it is deprecated and less secure.
Can I copy and paste complex formats like images with the Clipboard API?
Currently, the Clipboard API supports plain text. Support for other formats is limited.
What security considerations should I keep in mind?
The Clipboard API requires user interaction for access, reducing the risk of malicious use.
Is the Clipboard API available in all modern browsers?
Most modern browsers support the Clipboard API. Check for support to ensure compatibility.
Are there any libraries to simplify Clipboard API usage?
Yes, libraries like clipboard.js can simplify Clipboard API interactions.
Advanced Clipboard API Usage in JavaScript
Using the Clipboard API in advanced scenarios can unlock more powerful features for your web applications.
Through advanced handling, you can create more interactive and user-friendly features.
Here are some of the advanced methods and techniques to use with the Clipboard API.
Copying Rich Text and HTML to the Clipboard
Copying rich text or HTML involves using the ClipboardItem interface.
This method allows copying data other than plain text.
Here’s an example of copying HTML content to the clipboard:
// Function to copy HTML to clipboard
function copyHTMLToClipboard(html) {
const blob = new Blob([html], { type: 'text/html' });
const item = new ClipboardItem({ 'text/html': blob });
navigator.clipboard.write([item]).then(function() {
console.log('HTML copied to clipboard.');
}).catch(function(err) {
console.error('Failed to copy HTML.', err);
});
}
// Usage
copyHTMLToClipboard('Hello, World!');
This code demonstrates how to copy HTML to the clipboard using Blob and ClipboardItem.
The write() method is used to write the ClipboardItem to the clipboard.
Pasting HTML from the Clipboard
Pasting HTML content requires reading the clipboard content using the ClipboardItem interface.
Here’s how to read and paste HTML content from the clipboard:
// Function to read HTML from clipboard
function readHTMLFromClipboard() {
navigator.clipboard.read().then(function(data) {
for (let item of data) {
item.getType('text/html').then(function(blob) {
blob.text().then(function(html) {
console.log('HTML read from clipboard:', html);
document.body.innerHTML = html; // Example usage - paste HTML into the document body
});
});
}
}).catch(function(err) {
console.error('Failed to read HTML.', err);
});
}
// Usage
readHTMLFromClipboard();
This code reads HTML from the clipboard and updates the document body with the pasted HTML.
It uses the read() method to achieve this functionality.
Handling Clipboard Events
Handling clipboard events like copy, cut, and paste can be useful for custom functionalities.
Here’s an example of handling these events in JavaScript:
// Adding event listeners for clipboard events
document.addEventListener('copy', function(e) {
console.log('Copy event detected.');
});
document.addEventListener('cut', function(e) {
console.log('Cut event detected.');
});
document.addEventListener('paste', function(e) {
console.log('Paste event detected.');
});
This code snippet adds event listeners for clipboard events, logging messages to the console.
Custom Clipboard Formats
The Clipboard API allows the use of custom clipboard formats for more specialized use cases.
Here’s how to define and use a custom clipboard format:
// Function to copy custom data to clipboard
function copyCustomDataToClipboard(data) {
const blob = new Blob([data], { type: 'application/custom-format' });
const item = new ClipboardItem({ 'application/custom-format': blob });
navigator.clipboard.write([item]).then(function() {
console.log('Custom data copied to clipboard.');
}).catch(function(err) {
console.error('Failed to copy custom data.', err);
});
}
// Usage
copyCustomDataToClipboard('Custom format data');
This code demonstrates copying custom format data to the clipboard.
The application/custom-format MIME type is used in this case.
Creating a Fallback For Unsupported Browsers
As the Clipboard API is not supported in all browsers, it’s important to provide a fallback.
Here’s how to implement a fallback using a textarea element:
// Function to copy text to clipboard with fallback
function copyTextFallback(text) {
if (!navigator.clipboard) {
const textarea = document.createElement('textarea');
textarea.value = text;
document.body.appendChild(textarea);
textarea.select();
document.execCommand('copy');
document.body.removeChild(textarea);
console.log('Text copied using fallback method.');
return;
}
navigator.clipboard.writeText(text).then(function() {
console.log('Text successfully copied.');
}).catch(function(err) {
console.error('Failed to copy text.', err);
});
}
// Usage
copyTextFallback('Hello, fallback world!');
This function attempts to use the Clipboard API first, but falls back to using document.execCommand() for unsupported browsers.
Secure Context Requirement
The Clipboard API requires a secure context (HTTPS) due to security and privacy concerns.
This secure context ensures that clipboard operations are only executed in a trustable environment.
Common Issues and Solutions Continued
Issue: Copying and Pasting Broken Links
Broken links during copy-paste operations are often due to incorrect handling of relative paths.
When copying HTML, ensure that all links are absolute URLs or handle relative paths correctly in your application.
Issue: Clipboard Data Not Persisting
Persistence of clipboard data can be an issue in some scenarios due to browser settings or permissions.
Ensure clipboard permissions are granted and check for any browser-specific restrictions that may affect clipboard persistence.
Enhancing Security with Permissions API
You can enhance the security aspect of clipboard operations using the Permissions API.
Here’s how to request and check for ‘clipboard-read’ permission:
// Request clipboard-read permission
navigator.permissions.query({ name: 'clipboard-read' }).then(function(permissionStatus) {
console.log('clipboard-read permission state is', permissionStatus.state);
permissionStatus.onchange = function() {
console.log('clipboard-read permission state changed to', this.state);
};
});
This code snippet requests clipboard-read permission and monitors its state.
Frequently Asked Questions
How do I copy text in JavaScript without the Clipboard API?
You can use document.execCommand(‘copy’) but it is deprecated and less secure.
Can I copy and paste complex formats like images with the Clipboard API?
Currently, the Clipboard API supports plain text. Support for other formats is limited.
What security considerations should I keep in mind?
The Clipboard API requires user interaction for access, reducing the risk of malicious use.
Is the Clipboard API available in all modern browsers?
Most modern browsers support the Clipboard API. Check for support to ensure compatibility.
Are there any libraries to simplify Clipboard API usage?
Yes, libraries like clipboard.js can simplify Clipboard API interactions.
Shop more on Amazon