JavaScript Map vs. Object: When to Use
Published June 23, 2024 at 7:57 pm
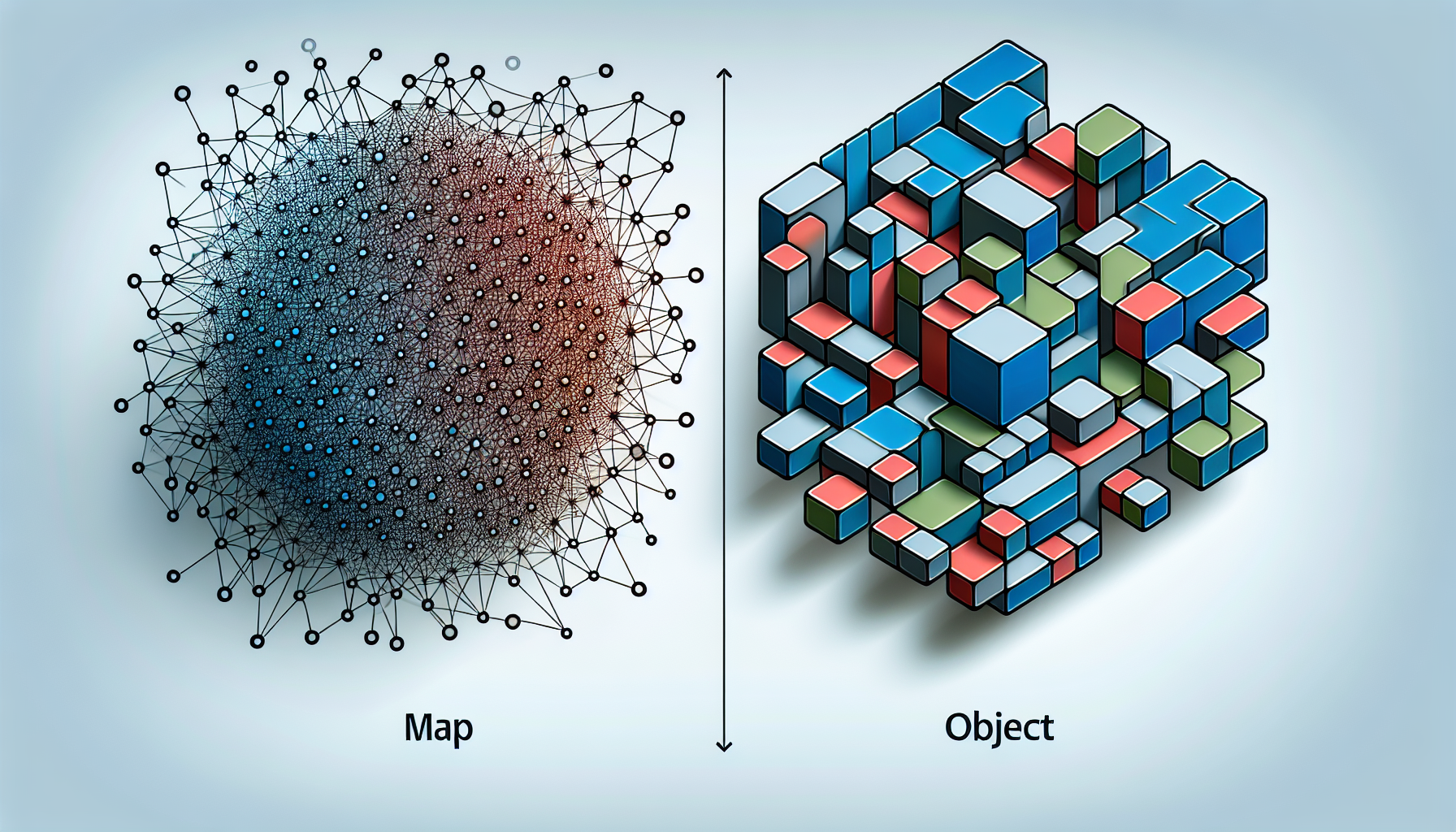
JavaScript Map vs. Object: When to Use
Choosing between a JavaScript Map and an Object might seem tricky, but the decision hinges on your specific needs.
TL;DR: When to Use Map vs. Object
Use a Map
if you need keys that are not strings, frequently add or remove pairs, or need better performance with large datasets.
Stick with an Object
if you need simple key-value pair structures with string keys, or methods like Object.keys()
, Object.values()
, and Object.entries()
.
Here is a practical example of using a Map
:
// Creating a Map
const userRoles = new Map([
['john', 'admin'],
['jane', 'editor'],
['dave', 'subscriber']
]);
// Accessing Map elements
console.log(userRoles.get('john')); // Output: 'admin'
// Adding and deleting elements in Map
userRoles.set('mike', 'guest');
userRoles.delete('dave');
console.log(userRoles.size); // Output: 3
Now lets explore using an Object
:
// Creating an Object
const userPermissions = {
john: 'admin',
jane: 'editor',
dave: 'subscriber'
};
// Accessing Object properties
console.log(userPermissions.john); // Output: 'admin'
// Adding and deleting properties in Object
userPermissions.mike = 'guest';
delete userPermissions.dave;
console.log(Object.keys(userPermissions).length); // Output: 3
Understanding the Basics: Map and Object
Both Maps and Objects serve the purpose of storing key-value pairs, but they do so in different ways.
An Object is part of JavaScript since its inception. It’s a fundamental data structure and can have keys which are strings or symbols.
A Map is a newer addition, introduced in ES6. Maps can have keys of any type, ensuring greater flexibility.
Performance Considerations
When performance is a concern, it’s important to recognize the strengths and weaknesses of both Maps and Objects.
Advantages of Map:
- Maps maintain the insertion order of keys.
- They allow for any datatype to be used as keys.
- Maps are optimized for frequent additions and deletions.
Advantages of Object:
- Objects are more lightweight for small datasets.
- They are highly compatible with JSON formats.
- Built-in methods like
Object.keys()
andObject.entries()
.
Use Case Scenarios
Deciding when to use a Map versus an Object often depends on the specific scenarios in your application.
Use a Map when:
- You need a key that is not a string.
- You require elements to maintain insertion order.
- You’re dealing with frequent additions and deletions.
Use an Object when:
- You have simple key-value pairs with string keys.
- You need compatibility with JSON.
- You need methods like
Object.keys()
,Object.values()
, andObject.entries()
.
Dealing with Large Datasets
When managing large datasets, efficiency and performance become critical.
Maps handle large datasets more efficiently due to their internal implementation, including faster average access times.
Objects can become cumbersome with large data due to their design for simpler key-value pairs and limited key type flexibility.
Initialization Differences
Initialization of Maps and Objects varies slightly and may influence your choice.
// Initializing a Map
const roles = new Map([
['admin', 'has full access'],
['editor', 'can edit content'],
['subscriber', 'can view content']
]);
// Initializing an Object
const permissions = {
admin: 'has full access',
editor: 'can edit content',
subscriber: 'can view content'
};
// Accessing elements
console.log(roles.get('admin')); // 'has full access'
console.log(permissions.admin); // 'has full access'
Methods and Properties
Maps provide:
map.set(key, value)
: Adds or updates key-value pairs.map.get(key)
: Retrieves the value associated with the key.map.delete(key)
: Removes the key-value pair by key.map.size
: Returns the number of key-value pairs.
Objects provide:
Object.keys(obj)
: Returns an array of property names.Object.values(obj)
: Returns an array of property values.Object.entries(obj)
: Returns an array of [key, value] pairs.
Common Issues and How to Fix Them
Let’s look at some common issues when choosing between Map and Object and how to address them.
Performance Bottlenecks:
Ensure you use Maps for large datasets with frequent updates to avoid performance hits.
Limit the use of Objects for large datasets to prevent sluggish performance.
Key Type Limitation:
Switch to using Maps if you are constrained by key type restrictions in Objects.
Use Maps to leverage any data type as a key.
Maintaining Key Order:
Use Maps to retain the key insertion order when iteration order matters.
Practical Implementations and Examples
It’s important to see real-world implementations to fully understand the benefits of using Maps or Objects.
// Example using Map
const productPrices = new Map([
['apple', 1.2],
['banana', 0.8],
['cherry', 2.5]
]);
console.log(productPrices.get('apple')); // 1.2
// Example using Object
const productStock = {
apple: 50,
banana: 100,
cherry: 25
};
console.log(productStock.apple); // 50
Handling Complex Data Structures
Complex data structures often require flexibility that Maps naturally provide.
When having complex keys, such as objects or arrays, Maps simplify management.
// Example with complex keys in Map
const users = new Map();
const user1 = { name: 'John' };
const user2 = { name: 'Jane' };
users.set(user1, 'admin');
users.set(user2, 'editor');
console.log(users.get(user1)); // 'admin'
Frequently Asked Questions
Q: Can I use Maps in all scenarios I use Objects?
You should use Maps when you need key flexibility. They offer advantages for complex key types.
Q: Do Maps provide better performance than Objects?
Yes, Maps are optimized for scenarios with frequent additions and deletions.
Q: Can I convert an Object to a Map?
Yes, use new Map(Object.entries(obj))
to convert an Object to a Map.
Q: Are there scenarios where Objects perform better?
Yes, Objects are more lightweight and ideal for small datasets where keys are strings.
Q: Do Maps maintain key order?
Absolutely, Maps retain the order of keys inserted which can be useful in ordered operations.
Q: What if I need JSON compatibility?
Use Objects for native JSON compatibility, ensuring seamless data interchange.
Wrapping Up the Comparison
Both Maps and Objects are essential tools in JavaScript, each with unique benefits.
Choose the one that best suits your needs based on key flexibility, dataset size, and operation types.
Exploring Map and Object Methods in Greater Depth
Both Maps and Objects come with a rich set of methods and properties that help manage and manipulate data.
Let’s delve deeper into some of these methods to fully understand their potential and use cases.
Map Methods and Properties:
map.set(key, value)
: Adds or updates key-value pairs.map.get(key)
: Retrieves the value associated with the key.map.delete(key)
: Removes the key-value pair by key.map.size
: Returns the number of key-value pairs.map.has(key)
: Checks if a key exists in the map.map.clear()
: Removes all key-value pairs from the map.
Object Methods and Properties:
Object.keys(obj)
: Returns an array of a given object’s property names.Object.values(obj)
: Returns an array of a given object’s property values.Object.entries(obj)
: Returns an array of the object’s own enumerable property [key, value] pairs.Object.assign(target, ...sources)
: Copies all enumerable own properties from one or more source objects to a target object.Object.create(proto, [propertiesObject])
: Creates a new object with the specified prototype object and properties.Object.freeze(obj)
: Freezes an object, making it immutable.
Deep Dive into Key Features
Diving deeper into the features of Maps and Objects helps in understanding their practical implications.
This aids in making an informed decision based on the specific needs of your application.
Insertion Order:
One key feature of Maps is that they retain the order of elements as they were inserted.
This is extremely useful when the sequence of data matters.
For example, you may want to maintain an order of user actions or events.
Iterable:
Both Maps and Objects can be iterated, but they differ in their approaches.
Maps are inherently iterable, allowing you to loop through key-value pairs directly using for...of
loops.
// Iterating through a Map
const fruits = new Map([
['apple', 1],
['banana', 2],
['cherry', 3]
]);
for (let [key, value] of fruits) {
console.log(key, value); // apple 1, banana 2, cherry 3
}
Objects, however, require additional methods like Object.keys()
, Object.values()
, or Object.entries()
for iteration.
// Iterating through an Object using Object.entries()
const vegetables = {
carrot: 1,
broccoli: 2,
spinach: 3
};
for (let [key, value] of Object.entries(vegetables)) {
console.log(key, value); // carrot 1, broccoli 2, spinach 3
}
Use Cases with Examples
Concrete examples make it easier to understand the usage of Maps and Objects.
Here’s how you can leverage these data structures in real-world scenarios.
Example: User Permissions with Maps
Let’s consider a scenario where user permissions in a web application are managed using a Map.
// Managing user permissions using Map
const userPermissions = new Map();
userPermissions.set('admin', ['add', 'edit', 'delete']);
userPermissions.set('editor', ['edit']);
userPermissions.set('viewer', ['view']);
console.log(userPermissions.get('admin')); // Output: ['add', 'edit', 'delete']
console.log(userPermissions.has('editor')); // Output: true
userPermissions.delete('viewer');
console.log(userPermissions.size); // Output: 2
Example: Product Inventory with Object
Let’s now look at managing a simple product inventory using an Object.
// Managing product inventory using Object
const productInventory = {
apple: { price: 1.2, stock: 100 },
banana: { price: 0.8, stock: 200 },
cherry: { price: 2.5, stock: 50 }
};
console.log(productInventory.apple.price); // Output: 1.2
// Updating stock
productInventory.banana.stock = 180;
delete productInventory.cherry;
console.log(Object.keys(productInventory).length); // Output: 2
Handling Complex Nested Structures
When dealing with nested data structures, your choice between Map and Object becomes more pivotal.
Each offers different degrees of flexibility and functionality for complex nesting.
Complex Nesting with Maps:
Maps can be nested within other Maps, providing a clear structure for complex data.
// Nested Maps for complex structures
const nestedMap = new Map();
const innerMap1 = new Map();
innerMap1.set('subKey1', 'value1');
innerMap1.set('subKey2', 'value2');
nestedMap.set('key1', innerMap1);
const innerMap2 = new Map();
innerMap2.set('subKeyA', 'valueA');
innerMap2.set('subKeyB', 'valueB');
nestedMap.set('key2', innerMap2);
console.log(nestedMap.get('key1').get('subKey1')); // Output: value1
Complex Nesting with Objects:
Objects can also be nested but may require more boilerplate code.
// Nested Objects for complex structures
const nestedObject = {
key1: {
subKey1: 'value1',
subKey2: 'value2'
},
key2: {
subKeyA: 'valueA',
subKeyB: 'valueB'
}
};
console.log(nestedObject.key1.subKey1); // Output: value1
Interoperability Considerations
When working with different systems or libraries, interoperability is a factor.
JSON is a common format for data interchange, which works seamlessly with Objects.
Using Objects with JSON:
Objects can be directly converted to JSON strings using JSON.stringify()
and parsed back with JSON.parse()
.
// Converting Object to JSON and back
const userSettings = {
theme: 'dark',
notifications: true,
language: 'en'
};
const jsonString = JSON.stringify(userSettings);
console.log(jsonString); // Output: JSON string
const parsedSettings = JSON.parse(jsonString);
console.log(parsedSettings.theme); // Output: dark
Best Practices and Recommendations
Consider the following best practices when deciding between Map and Object:
Use a Map if:
- You need keys that are not strings.
- Order of keys matters for your application.
- You anticipate frequent additions and deletions of key-value pairs.
Use an Object if:
- Compact syntax and native JSON support are priorities.
- You’re working with static, string-keyed data, especially in small datasets.
- You need to leverage built-in Object methods for property enumeration and manipulation.
Understanding Their Limitations
Knowing the limitations of both Maps and Objects helps you avoid pitfalls.
Map Limitations:
Maps, though powerful, can be overkill for small, simple datasets.
They do not convert directly to JSON, requiring manual transformations.
Object Limitations:
Objects can’t have non-string keys which limits flexibility.
They do not ensure key order when iterating, which can be problematic for ordered data.
Implementing Advanced Techniques
Advanced techniques can extend the functionality of both Maps and Objects.
Creating a Dynamic Map:
Maps can dynamically adjust keys and values, allowing for complex manipulations.
// Dynamic key manipulation in Map
const dynamicMap = new Map();
dynamicMap.set('item1', { name: 'Laptop', price: 1200 });
dynamicMap.set('item2', { name: 'Phone', price: 800 });
dynamicMap.forEach((value, key) => {
value.price *= 0.9; // Apply a discount to all items
});
console.log(dynamicMap.get('item1').price); // Output: 1080
Dynamic Property Definitions in Objects:
Objects can also dynamically define properties, offering flexible adjustments.
// Dynamic property manipulation in Object
const dynamicObject = {
product1: { name: 'Tablet', price: 300 },
product2: { name: 'Headphones', price: 150 }
};
for (let key in dynamicObject) {
dynamicObject[key].price *= 0.85; // Apply a discount to all products
}
console.log(dynamicObject.product1.price); // Output: 255
FAQs
Q: Can a Map hold multiple types of keys?
Yes, Maps can hold keys of any data type, such as strings, numbers, objects, etc.
Q: Are Objects always faster than Maps?
Not necessarily. While Objects are performant for small datasets, Maps can be faster for larger and more complex datasets.
Q: How can I convert a Map to an Array?
You can use the Array.from(map)
method to convert a Map to an Array.
Q: Is it possible to merge multiple Objects?
Yes, you can use Object.assign(target, ...sources)
to merge multiple objects into one.
Q: Can I iterate over Object properties directly?
Objects are not inherently iterable. Use methods like Object.keys()
, Object.values()
or Object.entries()
for iteration.
Q: Can Maps be serialized to JSON?
Maps cannot be directly serialized to JSON. Convert them to an Object or Array first.
Shop more on Amazon