Using PHP to Interact with Google Sheets API
Published February 20, 2024 at 8:00 am
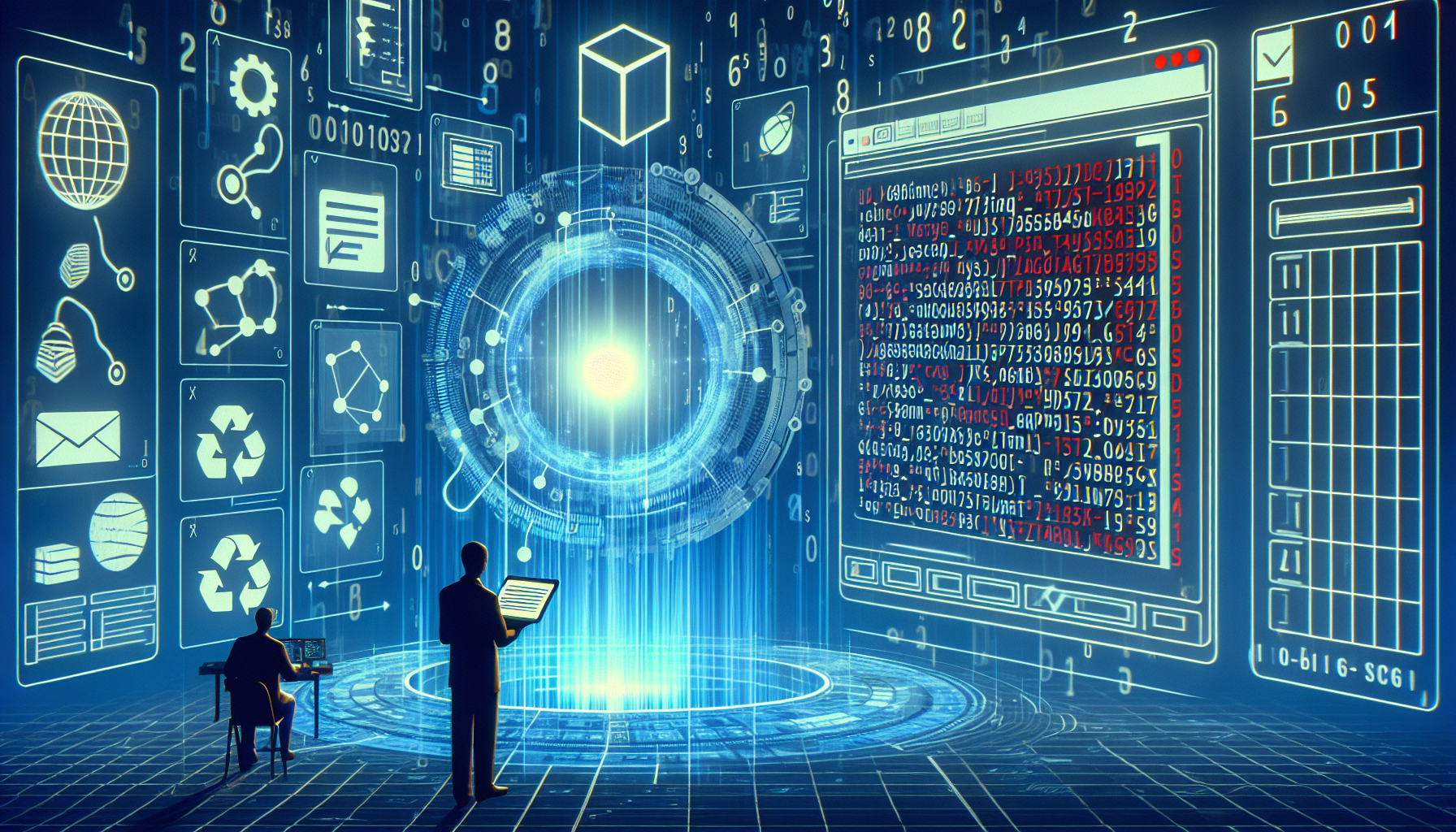
Understanding PHP and Google Sheets API Integration
If you are looking to harness the power of Google Sheets through your PHP application, you are at the right place.
This article will guide you through the process of using PHP to interact with the Google Sheets API.
TLDR: Quick Guide on PHP and Google Sheets API
The integration of PHP and Google Sheets API allows you to read from and write to Google Sheets programmatically.
You will need a Google Cloud Platform project, OAuth 2.0 credentials, and the Google Client Library for PHP.
Setting Up Your Google Cloud Project
Before any code can be executed, you must set up a project on the Google Cloud Platform (GCP).
This involves creating a new project, enabling the Sheets API, and setting up OAuth 2.0 credentials.
Installing Google Client Library for PHP
Use Composer to install the Google Client Library which simplifies the API communication.
The library offers methods to authenticate, create, and access Google Sheets with ease.
Authentication with OAuth 2.0
OAuth 2.0 is an authorization framework that allows your application to access Google Sheets on behalf of the user.
You will need to configure an OAuth consent screen and obtain the necessary client ID and client secret.
Accessing Google Sheets via PHP
With the setup complete, you are ready to access Google Sheets using PHP.
This involves obtaining an access token and making API requests to fetch or manipulate spreadsheet data.
Reading Data from Google Sheets
By specifying the sheet range, you can read data and bring it into your PHP application for further processing.
This is done using the `get` method provided by the Sheets API client instance.
Writing Data to Google Sheets
Adding or updating data in your Google Sheet is straightforward with the correct API call.
You can choose the range and utilize the `batchUpdate` or `update` function for these operations.
Error Handling and Best Practices
Robust error handling is crucial to ensure reliability when interacting with external APIs.
Set up try-catch blocks to gracefully manage exceptions and log errors for future debugging.
Advanced Features: Batch Requests and Conditional Formatting
For efficiency, learn to use batch requests which enable you to send multiple update commands in a single API call.
Explore conditional formatting rules to dynamically style your Google Sheets data via PHP logic.
Security Considerations
When dealing with APIs, especially with user data, it is essential to handle authentication tokens securely.
Ensure only the necessary permissions are granted and that tokens are stored and transmitted securely.
Pros and Cons of Using PHP with Google Sheets API
Pros
- Allows for automation of repetitive tasks related to data entry and management.
- Combines the robust functionality of Google Sheets with the flexibility of PHP.
- Supports both read and write operations, making it versatile for various applications.
Cons
- Requires initial setup time to understand and configure the Google Cloud Platform and OAuth consent.
- Dependent on Google’s API limitations and quotas, which might require careful handling for large-scale applications.
Common Issues and Troubleshooting
From permission errors to exceeded rate limits, several issues might arise when using the Google Sheets API with PHP.
Understanding these common pitfalls and how to address them is essential for smooth operation.
FAQs on Using PHP with Google Sheets API
How do I create a Google Cloud Platform project for accessing Google Sheets API?
You can create a project by going to the GCP Console, clicking ‘Create Project’, and following the on-screen instructions.
What is Composer and why do I need it for PHP Google Sheets API integration?
Composer is a tool for dependency management in PHP, allowing you to declare and install libraries your project requires.
Can I access Google Sheets without OAuth 2.0 in PHP?
No, you must use OAuth 2.0 for authorization as it ensures secure access to the user’s spreadsheet data.
What are rate limits and quotas in the context of Google API?
Rate limits and quotas are restrictions imposed by Google to prevent abuse and overload of their services.
How can I handle large datasets with Google Sheets API and PHP without exceeding quotas?
Optimize your API requests, utilize batch requests, and consider implementing exponential backoff for retry mechanisms.
Example of Reading Data from a Google Sheet
First, authenticate with Google Client and access the Sheets service.
Then, specify the spreadsheet ID and the range of cells you wish to retrieve.
<?php
require 'vendor/autoload.php';
$client = new \Google_Client();
$client->setApplicationName('Google Sheets and PHP Integration');
$client->setScopes([\Google_Service_Sheets::SPREADSHEETS]);
$client->setAccessType('offline');
$client->setAuthConfig(__DIR__ . '/credentials.json');
$service = new Google_Service_Sheets($client);
$spreadsheetId = 'your-spreadsheet-id';
$range = 'Sheet1!A2:E10';
$response = $service->spreadsheets_values->get($spreadsheetId, $range);
$values = $response->getValues();
?>
This code snippet fetches the data from the specified range of your Google Sheet.
Example of Writing Data to a Google Sheet
To update a Google Sheet, define the values you want to write and specify the range.
<?php
// Other initialization code...
$body = new Google_Service_Sheets_ValueRange([
'values' => [['Data 1', 'Data 2', 'Data 3']] // Row data to insert
]);
$params = ['valueInputOption' => 'RAW'];
$range = 'Sheet1!A2:C2';
$updateResult = $service->spreadsheets_values->update($spreadsheetId, $range, $body, $params);
?>
This script will insert data into your specified range in the Google Sheet.
Conclusion
In summary, integrating PHP with Google Sheets API opens a world of possibilities for application development.
With careful setup, understanding of Google’s API constraints, and proper coding practices, integrating the two can be a smooth process enriching your apps with the powerful features of Google Sheets.
How to Set Up OAuth Consent Screen for Google Sheets API
After creating a Google Cloud Platform project, setting up an OAuth consent screen is a vital step.
This screen provides information to users about the app requesting their data and what type of access it requires.
Retrieving and Storing Google Sheets API Tokens Safely
Upon gaining user consent, you will receive tokens which need secure handling and storage.
Consider environment variables or secure database storage, and refresh them periodically for continued access.
Efficiently Managing Google API Rate Limits
Bumping into API rate limits can be a common nuisance while working with Google Sheets and PHP.
To mitigate this, implement efficient cashing, stagger your requests, and heed Google’s best practices and guidelines.
Boosting Performance with Batch API Calls
Minimizing the number of API calls can profoundly improve performance when working with large datasets.
Learn to combine multiple API calls into a batch request, thus reducing network latency and resource consumption.
Implementing Conditional Formatting with PHP
Conditional formatting rules can alter the appearance of your Google Sheets data based on specific conditions.
You can define and apply these rules within your PHP code to make your Sheets dynamic and visually engaging.
Maintaining Security and Privacy Compliance
Handling data comes with responsibility and requires adherence to privacy laws and regulations.
Maintain an up-to-date understanding of compliance requirements, and implement strict access controls.
Expanding Functionality with Google Sheets API Add-Ons
Beyond native API functionalities, consider utilizing add-ons available in the G Suite Marketplace for expanded capabilities.
They can streamline workflows and add advanced features to your projects.
Getting Support and Resources for Troubleshooting
Joining developer forums, reading official documentation, and utilizing Stack Overflow can be invaluable for overcoming obstacles.
Leverage these resources when troubleshooting issues during your PHP and Google Sheets API integration.
Testing and Validating Your PHP Google Sheets Integration
Quality assurance plays a crucial role in the integration process.
Use testing frameworks and validation tools to ensure your code runs correctly and the data is handled as expected.
Exploring New Google Sheets API Features for PHP Developers
Google is continuously updating its APIs, providing new opportunities for development and innovation.
Keep an eye out for updates and experiment with new features to maintain a cutting-edge application.
FAQs on Using PHP with Google Sheets API
How do I handle API errors in PHP when interacting with Google Sheets?
Utilize try-catch blocks to catch exceptions, check error responses from the API, and implement a logging mechanism for errors.
What should I do if my application needs to access multiple Google Sheets?
You can access multiple sheets by specifying different spreadsheet IDs while making the API requests, ensuring your app has the requisite permissions.
Are there any alternatives to using OAuth 2.0 for server-to-server interactions?
For server-to-server communications, you can use a service account with its own credentials, which does not require user consent for accessing Google Sheets.
Is it possible to set up webhooks with Google Sheets and PHP?
Google Sheets API does not support webhooks directly, but you can set up polling or use Google Apps Script to trigger actions on changes.
How do I optimize my Google Sheets API usage to stay within free usage limits?
To stay within the free usage limits, cache responses when possible, avoid unnecessary calls by checking for actual data changes, and batch requests whenever applicable.
Common Issues and How to Troubleshoot Them
When integrating PHP with Google Sheets API, you might encounter various issues that can disrupt your workflow.
Identifying these issues early and knowing how to troubleshoot them can save you from potential headaches and downtime.
Lets explore some frequent challenges developers face, along with troubleshooting steps to resolve them promptly.
One issue you might come across is receiving an ‘invalid_grant’ error.
This usually indicates a problem with the OAuth 2.0 credentials.
To fix this, ensure that the time on your server is synchronized with NTP and the credentials are set up correctly in your Google Cloud Platform project.
Another common obstacle is exceeding Google API rate limits, resulting in ‘quota_exceeded’ errors.
Address this by optimizing your API call patterns, implementing retries with exponential back-off, and reviewing your usage in the Google Cloud Platform Console to potentially request an increase in your quota.
Occasionally, your app may experience intermittent ‘500’ and ‘503’ errors, suggesting issues on Google’s end.
In such cases, implementing robust retry logic in your application can help manage these transient errors smoothly.
Authentication and Permission Issues: When your app suddenly cannot access a Google Sheet, it might be due to revoked permissions or expired tokens.
Ensure your application regularly refreshes access tokens using the refresh token, and always check for changes in permission settings for the Google Sheets in question.
In instances where data is not being written accurately to Google Sheets, double-check the range and values specified in your update requests.
Miscalculations in specifying cell ranges or incorrect data formats can often lead to unexpected outcomes.
Last but not least, you might experience difficulties setting up the correct scopes for your application.
Scopes define the level of access your app has to Google Sheets data.
Confirm that the scopes defined in your PHP application match those enabled in your Google Cloud Platform project.
Addressing these common issues involves a combination of careful planning, understanding potential error messages, and smart coding practices.
By implementing the above best practices, you can significantly minimize disruptions in your PHP and Google Sheets API integration.
Shop more on Amazon